It is easy to reverse the order of rows in a Pandas DataFrame
In data analysis and manipulation, it is common to encounter situations where you need to reverse the order of rows in a Pandas DataFrame. Reversing the order of rows can be useful for various tasks, such as examining data from a different perspective or preparing the data for specific analysis requirements.
In this tutorial, we will explore different methods to reverse the order of rows in a Pandas DataFrame using a dataset sourced from a provided URL. We will walk through step-by-step instructions to help you accomplish this task using slicing
, reindex
,.iloc indexer
sort_index
and sort_values
methods in Pandas.
1. Read Dataset
For the purpose of this tutorial, let’s use a dataset apple_share_price.csv
in a GitHub repository.
# import the necessary libraries
import pandas as pd
# Read the dataset from the provided URL into a DataFrame
url = 'https://raw.githubusercontent.com/NourozR/Stock-Price-Prediction-LSTM/master/apple_share_price.csv'
df = pd.read_csv(url)
# display the DataFrame
df
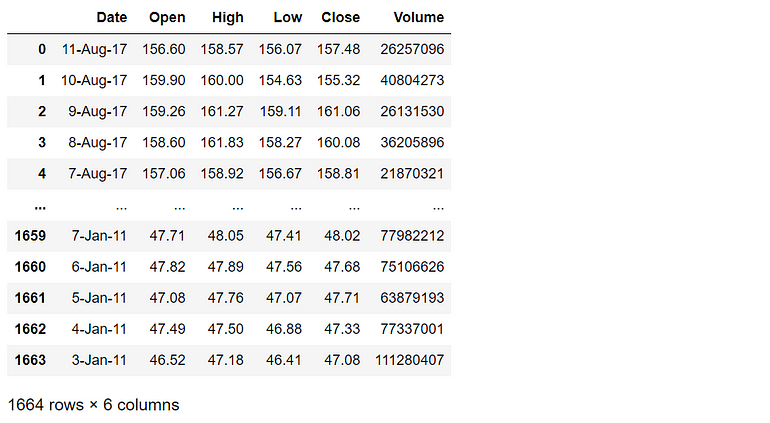
From the above result, we can see that the dataset is reverse-chronological. In the following examples, we reverse its order.
2. Slicing Method
We can easily reverse the order of DataFrame using slicing method as follows.
df_reversed = df[::-1]
df_reversed
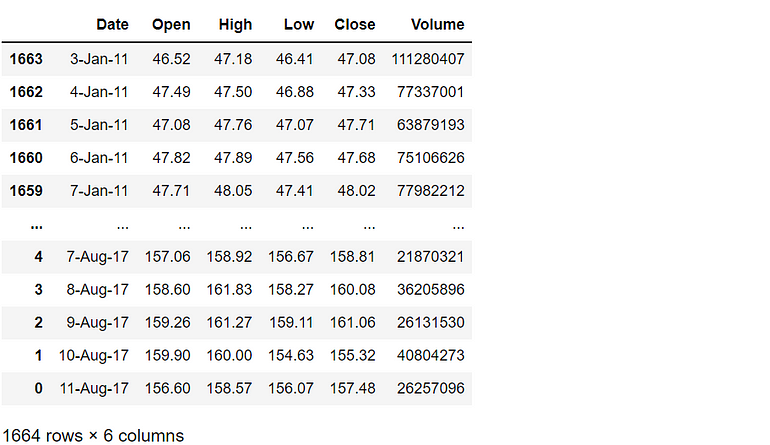
You can see that the index is also reversed, but we can use reset_index
to make it in chronological order.
df_reversed = df[::-1].reset_index(drop=True)
df_reversed

The [::-1] slicing notation is used to reverse the order of rows. The reset_index(drop=True)
function is called to reset the index of the reversed DataFrame.
3. Reindex Method
Instead of using slicing
, you can achieve the same result by using the reindex
function in Pandas. Here’s an updated version of the code using reindex
:
df_reversed = df.reindex(index=df.index[::-1]).reset_index(drop=True)
df_reversed

In this code, we use the reindex
function with the reversed index of the DataFrame (df.index[::-1]
). This will reorder the rows of the DataFrame in reverse order. The reset_index(drop=True)
function is then used to reset the index of the reversed DataFrame.
Running this updated code will produce the same result as the previous code, with the rows in the DataFrame reversed.
4. Indexer Method
You can also use the .iloc
indexer with slicing to reverse the order of rows in a Pandas DataFrame. Here’s the updated code using .iloc[::-1]
:
df_reversed = df.iloc[::-1].reset_index(drop=True)
df_reversed

In this code, df.iloc[::-1]
is used to retrieve the rows in reverse order. The reset_index(drop=True)
function is then used to reset the index of the reversed DataFrame.
Running this updated code will produce the same result as before, with the rows of the DataFrame reversed.
5. Sort Index Method
You can also use the sort_index
function to reverse the order of rows in a Pandas DataFrame. Here’s the updated code using sort_index
:
df_reversed = df.sort_index(ascending=False).reset_index(drop=True)
df_reversed

Besides, you can also use ignore_index=True
to reach the same result as reset_index(drop=True)
with the following snippet.
df_reversed = df.sort_index(ascending=False,ignore_index=True)<br>df_reversed
6. Sort Value Method
You can also use the sort_values
function to reverse the order of rows in a Pandas DataFrame. Here’s the updated code using sort_values
. We convert the Date
to time objective first.
df['Date'] = pd.to_datetime(df['Date'],format='%Y-%m-%d')<br><br>df_reversed = df.sort_values(by=['Date'], ascending=True).reset_index(drop=True)<br>df_reversed

In this code, df.sort_values(by=df.index, ascending=False)
is used to sort the DataFrame by the index in descending order, effectively reversing the order of rows. The reset_index(drop=True)
function is then used to reset the index of the reversed DataFrame.
Running this updated code will produce the same result as before, with the rows of the DataFrame reversed.
Conclusion
Reversing the order of rows in a Pandas DataFrame is a straightforward operation that can be achieved using multiple approaches. In this tutorial, we explored five methods: slicing
, reindex
,.iloc indexer
sort_index
and sort_values
. Each method allows you to reverse the order of rows effectively, providing flexibility based on your preference and specific data analysis requirements. By mastering these techniques, you can confidently manipulate and transform DataFrame structures to suit your needs. Now that you have learned how to reverse the order of rows in a Pandas DataFrame, you can apply this knowledge to various data analysis tasks and further enhance your data manipulation skills.
Originally published at https://medium.com/@shouke.wei on June 20, 2023.