Displays 2 methods to rearrange a column of Pandas DataFrame
In data analysis and manipulation tasks, it is often necessary to rearrange the columns of a Pandas DataFrame to suit our needs. Whether we want to reorder columns for better readability, group related variables together, or perform specific calculations, Pandas provides several methods to easily move columns within a DataFrame. In this tutorial, we will explore two commonly used approaches: using the reindex()
method and the pop()
method.
Read Dataset
First, we read a well-know real-world dataset of iris from the UCI Machine Learning Repository.
import pandas as pd
url = 'https://archive.ics.uci.edu/ml/machine-learning-databases/iris/iris.data'
cols = ['sepal_length', 'sepal_width', 'petal_length', 'petal_width', 'class']
df = pd.read_csv(url, header=None, names=cols)
# print original dataframe<br>print("Original Dataframe")
display(df)
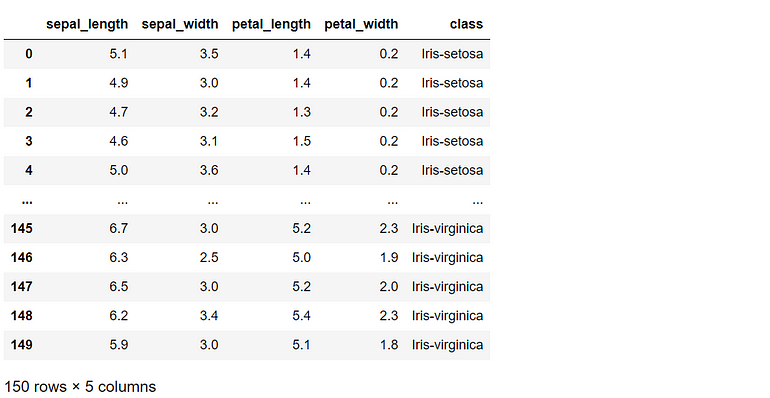
Move a column using reindex()
The reindex()
method can be used to move a column to a specific position. Here’s an example of moving column ‘petal_length’ to the second position:
df = df.reindex(columns=['sepal_length', 'petal_length', 'sepal_width', 'petal_width', 'class'])
df
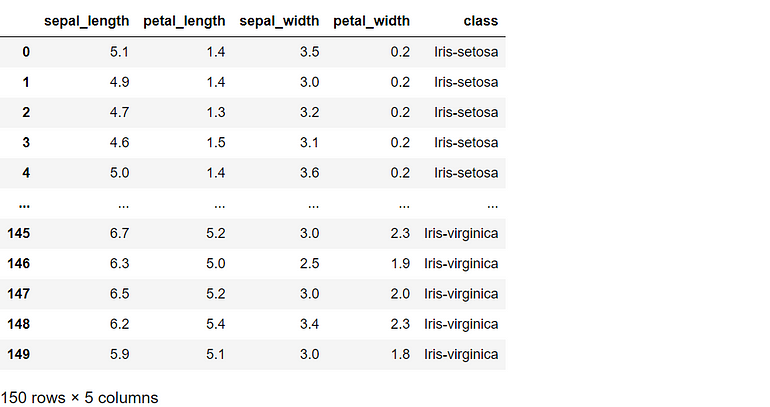
In this example, we provided the desired order of the columns as a list to the columns parameter of the reindex() method.
Move a column using pop()
The pop()
method can be used to move a column and remove it from the DataFrame. Here’s an example of moving column ‘class’ to the first position:
# extract column 'class' from the DataFrame
column_extract = df.pop('class')
# insert column using insert(position,column_name)
# for example, first_column
df.insert(0, 'class', column_extract)
df
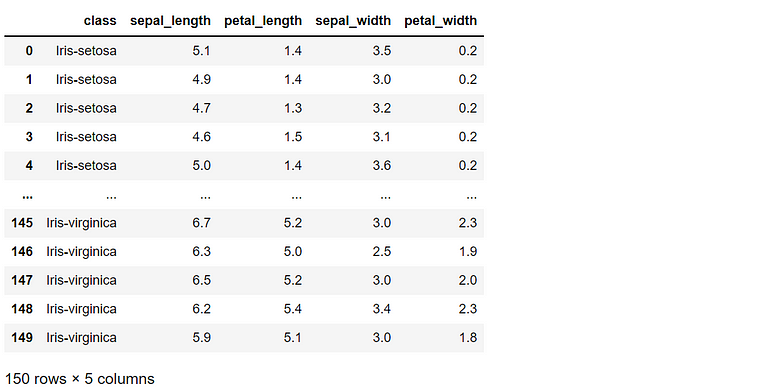
In this example, we used the pop()
method to extract column ‘class’ from the DataFrame and stored it in a separate variable. Then, we used the insert()
method to insert the column at the desired position (in this case, the first position).
Conclusion
In this tutorial, we learned how to move columns within a Pandas DataFrame. We explored two methods: reindex()
and pop()
. The reindex()
method allows us to specify a desired order for the columns by providing a list, while the pop()
method enables us to extract a column, remove it from the DataFrame, and insert it at a desired position using the insert()
method.
By mastering these techniques, you can efficiently rearrange columns in a Pandas DataFrame to suit your analysis or visualization needs. Whether you’re reordering columns for better readability, conducting specific calculations, or organizing related variables, these methods provide flexibility and control over your data manipulation tasks. Pandas offers a wide range of functionality, and knowing how to move columns is a valuable skill for any data analyst or scientist working with tabular data.
Originally published at https://medium.com/@shouke.wei on June 12, 2023.